本文將詳細(xì)介紹如何使用 C# 在 SQLite 數(shù)據(jù)庫(kù)中執(zhí)行 SELECT 操作。SELECT 操作是數(shù)據(jù)庫(kù)查詢中最常用和最重要的操作,用于從數(shù)據(jù)庫(kù)中檢索數(shù)據(jù)。
準(zhǔn)備工作
首先,確保你的項(xiàng)目中已安裝 System.Data.SQLite
NuGet 包。在你的 C# 文件頂部添加以下 using 語(yǔ)句:
using System;
using System.Data;
using System.Data.SQLite;
連接到數(shù)據(jù)庫(kù)
在執(zhí)行任何查詢之前,我們需要建立與數(shù)據(jù)庫(kù)的連接。以下是一個(gè)建立連接的輔助方法:
public static SQLiteConnection ConnectToDatabase(string dbPath)
{
try
{
SQLiteConnection connection = new SQLiteConnection($"Data Source={dbPath};Version=3;");
connection.Open();
return connection;
}
catch (Exception ex)
{
Console.WriteLine($"連接數(shù)據(jù)庫(kù)時(shí)出錯(cuò):{ex.Message}");
return null;
}
}
基本的 SELECT 操作
查詢所有記錄
以下是一個(gè)查詢表中所有記錄的方法:
public static void SelectAllRecords(SQLiteConnection connection, string tableName)
{
try
{
string sql = $"SELECT * FROM {tableName}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"查詢所有記錄時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
SelectAllRecords(connection, "Users");
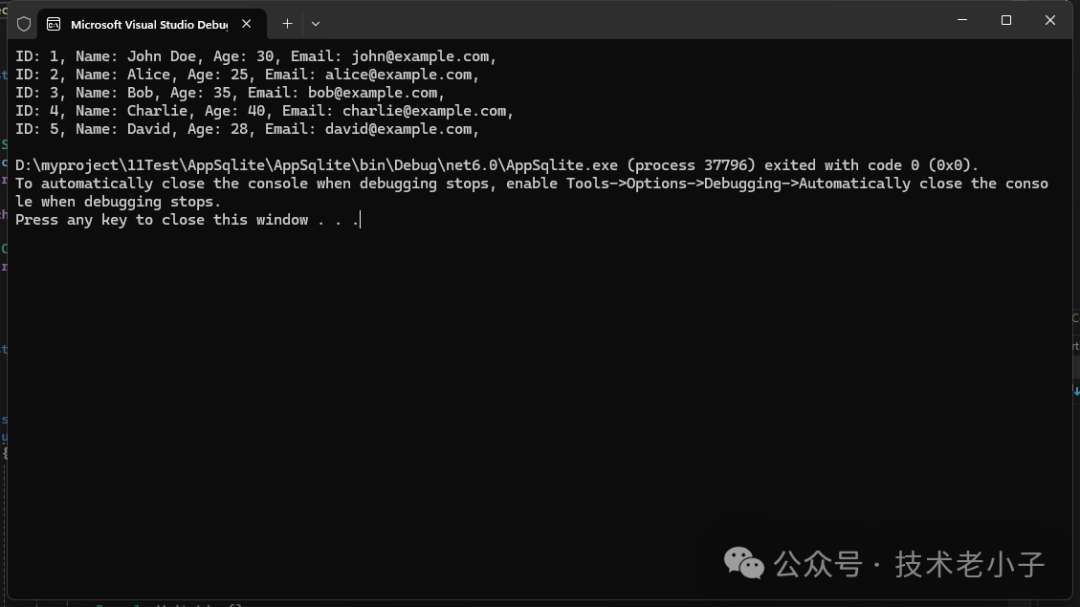
使用條件查詢
以下是一個(gè)帶條件的查詢方法:
public static void SelectRecordsWithCondition(SQLiteConnection connection, string tableName, string condition)
{
try
{
string sql = $"SELECT * FROM {tableName} WHERE {condition}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"條件查詢記錄時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
SelectRecordsWithCondition(connection, "Users", "Age > 30");
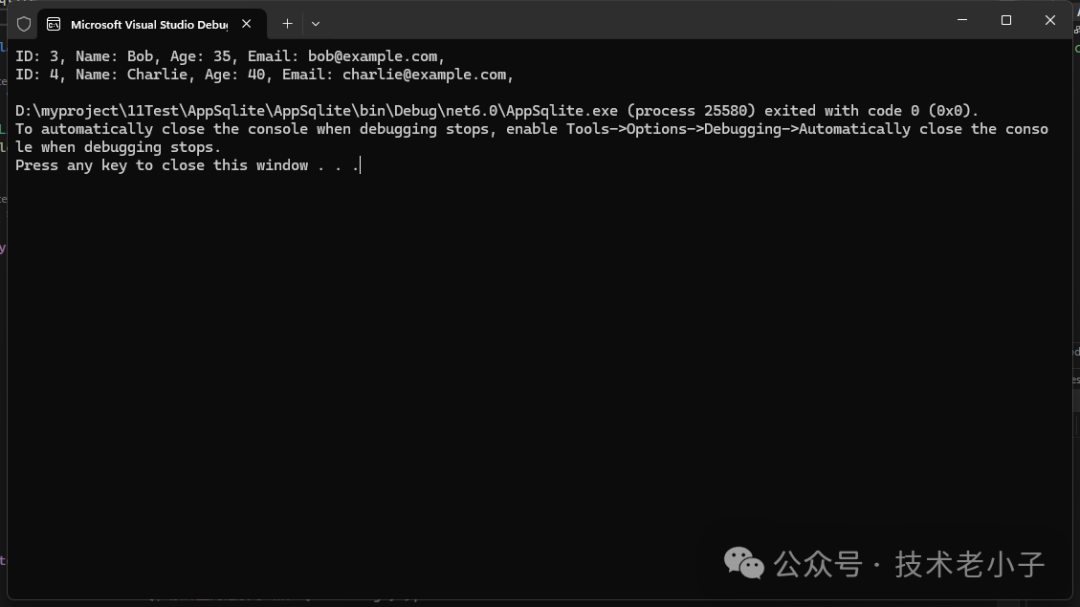
使用參數(shù)化查詢
為了防止 SQL 注入攻擊并提高性能,我們應(yīng)該使用參數(shù)化查詢:
public static void SelectRecordsWithParameters(SQLiteConnection connection, string tableName, string condition, Dictionary<string, object> parameters)
{
try
{
string sql = $"SELECT * FROM {tableName} WHERE {condition}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
foreach (var param in parameters)
{
command.Parameters.AddWithValue(param.Key, param.Value);
}
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"參數(shù)化查詢記錄時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
var parameters = new Dictionary<string, object>
{
{ "@minAge", 25 },
{ "@maxAge", 40 }
};
SelectRecordsWithParameters(connection, "Users", "Age BETWEEN @minAge AND @maxAge", parameters);
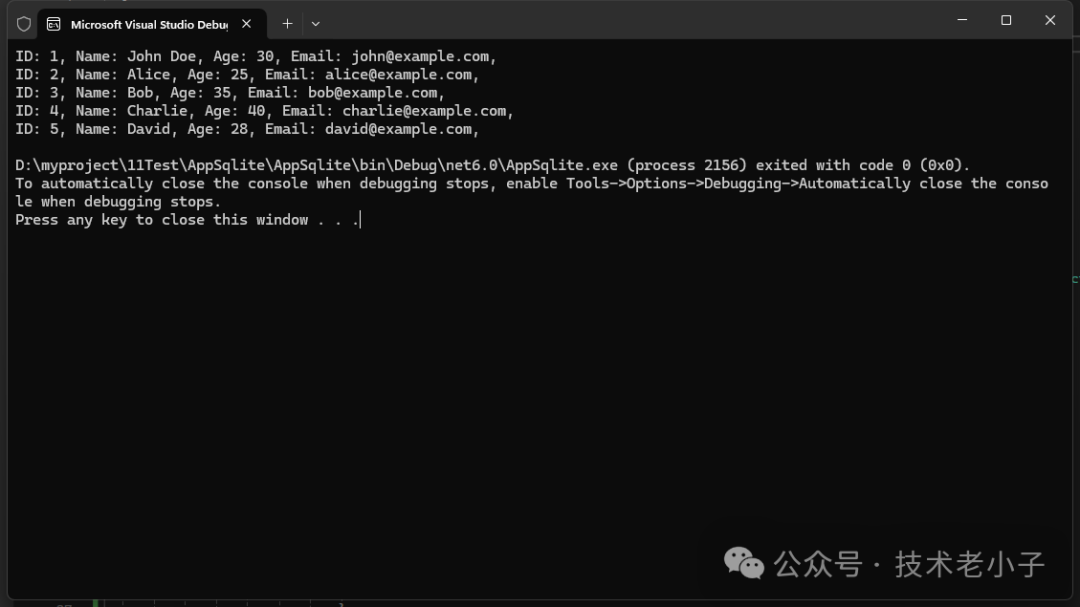
高級(jí) SELECT 操作
使用聚合函數(shù)
SQLite 支持多種聚合函數(shù),如 COUNT, AVG, SUM 等。以下是一個(gè)使用聚合函數(shù)的示例:
public static void SelectWithAggregation(SQLiteConnection connection, string tableName, string aggregation)
{
try
{
string sql = $"SELECT {aggregation} FROM {tableName}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
object result = command.ExecuteScalar();
Console.WriteLine($"聚合結(jié)果:{result}");
}
}
catch (Exception ex)
{
Console.WriteLine($"執(zhí)行聚合查詢時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
SelectWithAggregation(connection, "Users", "AVG(Age)");
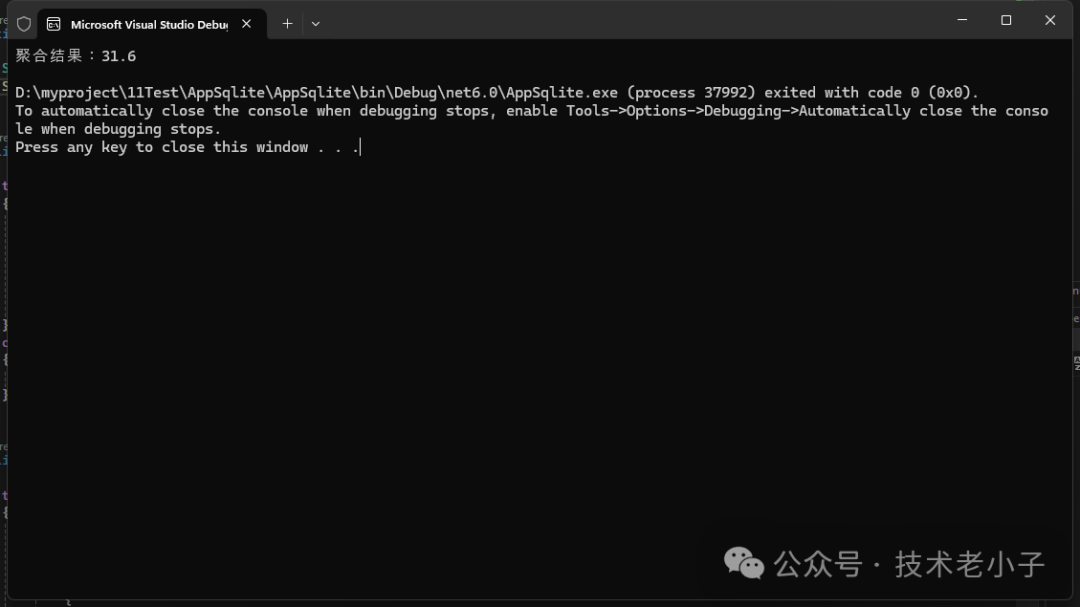
使用 ORDER BY 和 LIMIT
以下是一個(gè)帶排序和限制結(jié)果數(shù)量的查詢方法:
public static void SelectWithOrderAndLimit(SQLiteConnection connection, string tableName, string orderBy, int limit)
{
try
{
string sql = $"SELECT * FROM {tableName} ORDER BY {orderBy} LIMIT {limit}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"執(zhí)行排序和限制查詢時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
SelectWithOrderAndLimit(connection, "Users", "Age DESC", 5);
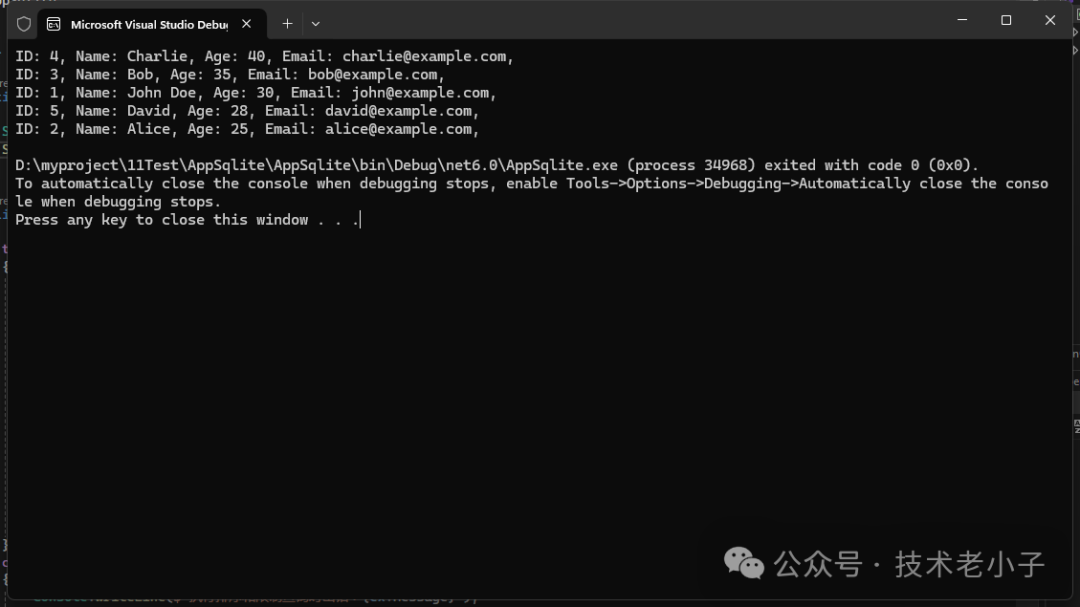
使用 JOIN
以下是一個(gè)使用 JOIN 的示例方法:
public static void SelectWithJoin(SQLiteConnection connection, string table1, string table2, string joinCondition)
{
try
{
string sql = $"SELECT * FROM {table1} INNER JOIN {table2} ON {joinCondition}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write($"{reader.GetName(i)}: {reader[i]}, ");
}
Console.WriteLine();
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"執(zhí)行 JOIN 查詢時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
SelectWithJoin(connection, "Users", "Orders", "Users.Id = Orders.UserId");
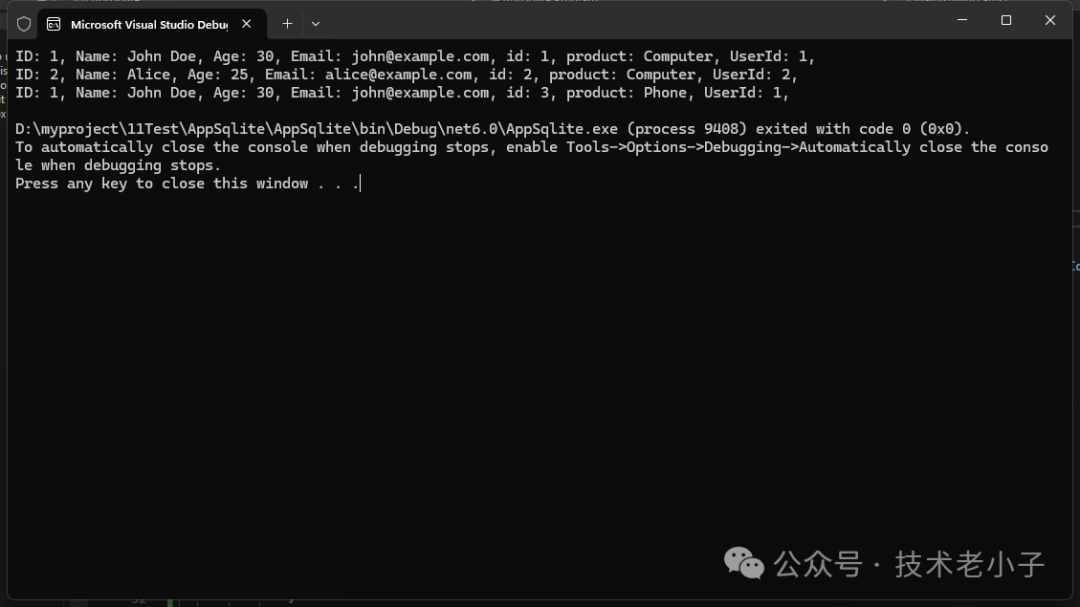
使用 DataTable 存儲(chǔ)結(jié)果
對(duì)于需要在內(nèi)存中處理查詢結(jié)果的情況,我們可以使用 DataTable:
public static DataTable SelectToDataTable(SQLiteConnection connection, string sql)
{
try
{
DataTable dt = new DataTable();
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
using (SQLiteDataAdapter adapter = new SQLiteDataAdapter(command))
{
adapter.Fill(dt);
}
}
return dt;
}
catch (Exception ex)
{
Console.WriteLine($"查詢到 DataTable 時(shí)出錯(cuò):{ex.Message}");
return null;
}
}
使用示例:
DataTable dt = SelectToDataTable(connection, "SELECT * FROM Users WHERE Age > 30");
foreach (DataRow row in dt.Rows)
{
foreach (DataColumn col in dt.Columns)
{
Console.Write($"{col.ColumnName}: {row[col]}, ");
}
Console.WriteLine();
}
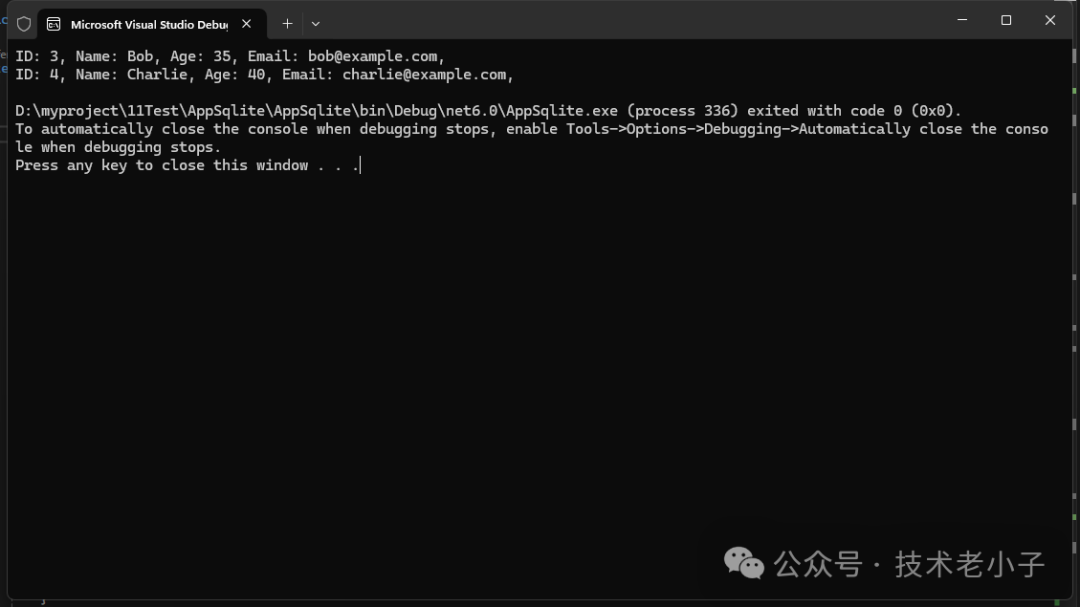
完整示例
以下是一個(gè)完整的示例,展示了如何使用上述所有方法:
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.SQLite;
class Program
{
static void Main(string[] args)
{
string dbPath = "C:\\example.db";
SQLiteConnection connection = ConnectToDatabase(dbPath);
if (connection != null)
{
// 查詢所有記錄
Console.WriteLine("所有用戶:");
SelectAllRecords(connection, "Users");
// 條件查詢
Console.WriteLine("\n30歲以上的用戶:");
SelectRecordsWithCondition(connection, "Users", "Age > 30");
// 參數(shù)化查詢
Console.WriteLine("\n25到40歲的用戶:");
var parameters = new Dictionary<string, object>
{
{ "@minAge", 25 },
{ "@maxAge", 40 }
};
SelectRecordsWithParameters(connection, "Users", "Age BETWEEN @minAge AND @maxAge", parameters);
// 聚合查詢
Console.WriteLine("\n用戶平均年齡:");
SelectWithAggregation(connection, "Users", "AVG(Age)");
// 排序和限制結(jié)果
Console.WriteLine("\n年齡最大的5個(gè)用戶:");
SelectWithOrderAndLimit(connection, "Users", "Age DESC", 5);
// JOIN 查詢
Console.WriteLine("\n用戶及其訂單:");
SelectWithJoin(connection, "Users", "Orders", "Users.Id = Orders.UserId");
// 查詢到 DataTable
Console.WriteLine("\n30歲以上的用戶(使用 DataTable):");
DataTable dt = SelectToDataTable(connection, "SELECT * FROM Users WHERE Age > 30");
foreach (DataRow row in dt.Rows)
{
foreach (DataColumn col in dt.Columns)
{
Console.Write($"{col.ColumnName}: {row[col]}, ");
}
Console.WriteLine();
}
// 關(guān)閉連接
connection.Close();
}
}
// 在這里實(shí)現(xiàn)所有上述方法
}
注意事項(xiàng)
始終使用參數(shù)化查詢來(lái)防止 SQL 注入攻擊。
對(duì)于大型結(jié)果集,考慮使用分頁(yè)查詢或流式處理來(lái)避免內(nèi)存問(wèn)題。
正確處理 NULL 值,特別是在使用聚合函數(shù)時(shí)。
在完成操作后,記得關(guān)閉數(shù)據(jù)庫(kù)連接。
對(duì)于頻繁執(zhí)行的查詢,可以考慮使用預(yù)處理語(yǔ)句(prepared statements)來(lái)提高性能。
結(jié)論
本文詳細(xì)介紹了如何使用 C# 在 SQLite 數(shù)據(jù)庫(kù)中執(zhí)行各種 SELECT 操作,包括基本查詢、條件查詢、參數(shù)化查詢、聚合查詢、排序和限制結(jié)果、JOIN 操作以及使用 DataTable 存儲(chǔ)結(jié)果。這些操作覆蓋了大多數(shù)常見(jiàn)的數(shù)據(jù)檢索場(chǎng)景。
該文章在 2024/10/22 12:24:35 編輯過(guò)